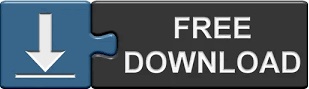

Mistake # 10: Using mutexes when std::atomic types will suffice A superficial solution might be to just use a std::recursive_mutex - but this is almost always indicative of a bad design.
#Qt update gui from different thread code
The fix is to structure your code in such a way that it does not try to acquire a previously locked mutex. Std::this_thread::sleep_for(std::chrono::milliseconds(100)) Here's a code snippet if you want to try to simulate a deadlock: #include "stdafx.h" In other words, your program is hanged at this point. Thread 1 cannot release lock A unless it has acquired lock B and so on. And from Thread 2's perspective, it is blocked on acquiring lock A, but cannot do so because Thread 1 is holding lock A. In some situations, what's going to happen is that when Thread 1 tries to acquire Lock B, it gets blocked because Thread 2 is already holding lock B.

This is one of the most common causes of DEADLOCK, a situation where threads block indefinitely because they are waiting to acquire access to resources currently locked by other blocked threads. Mistake # 8 : Not acquiring multiple locks in the same order }// lock_guard object is destroyed and mutex mu is released #include "stdafx.h"Ĭout lock(mu) // Start of Critical Section - to protect std::coutĬout << "Thread " << this_thread::get_id() << " says " << message << endl In the example below, we forgot to join t1 to the main thread. If we forgot to join a thread or detach it(make it unjoinable) before the main program terminates, it'll cause in a program crash. Mistake # 1: Not using join() to wait for background threads before terminating an application If you know any more pitfalls, or have alternative suggestions for some of the mistakes – please leave a comment below and I'll factor them into the article. In this article, I've tried to catalog all the mistakes I know of, with potential solutions.
#Qt update gui from different thread Patch
Most of these mistakes were luckily caught in code review and testing however, some arcane ones did slip through and make it into production code and we had to patch live systems, which is always expensive. I've made a number of mistakes myself over the years. Threading is one of the most complicated things to get right in programming, especially in C++.
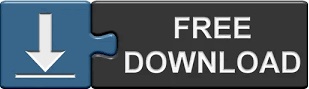